基于C#的蓝牙串口模块上位机开发
一、说明
本文章讨论使用C#,使个人主机与蓝牙串口模块进行通信。
硬件准备:
- 蓝牙串口模块(本次使用HC-04)
- CH340(USB转TTL)
软件准备:
- Visual Studio(安装C#.NET Framework框架)
- 串口调试助手(微软商店可下载)
操作系统:win10
功能:通过上位机向蓝牙模块发送数据
蓝牙协议:BLE4.0
二、创建工程
选择C# 控制台应用(.NET Framework)
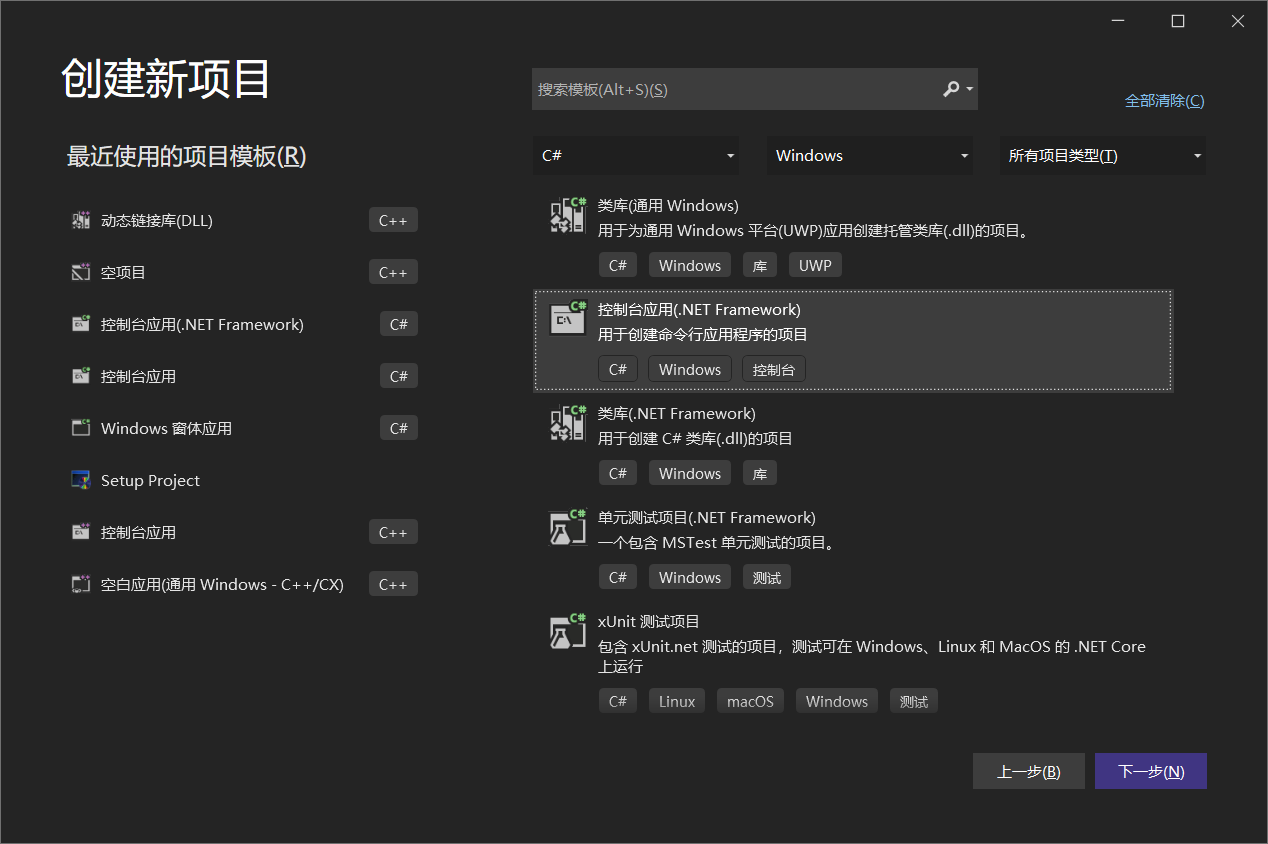
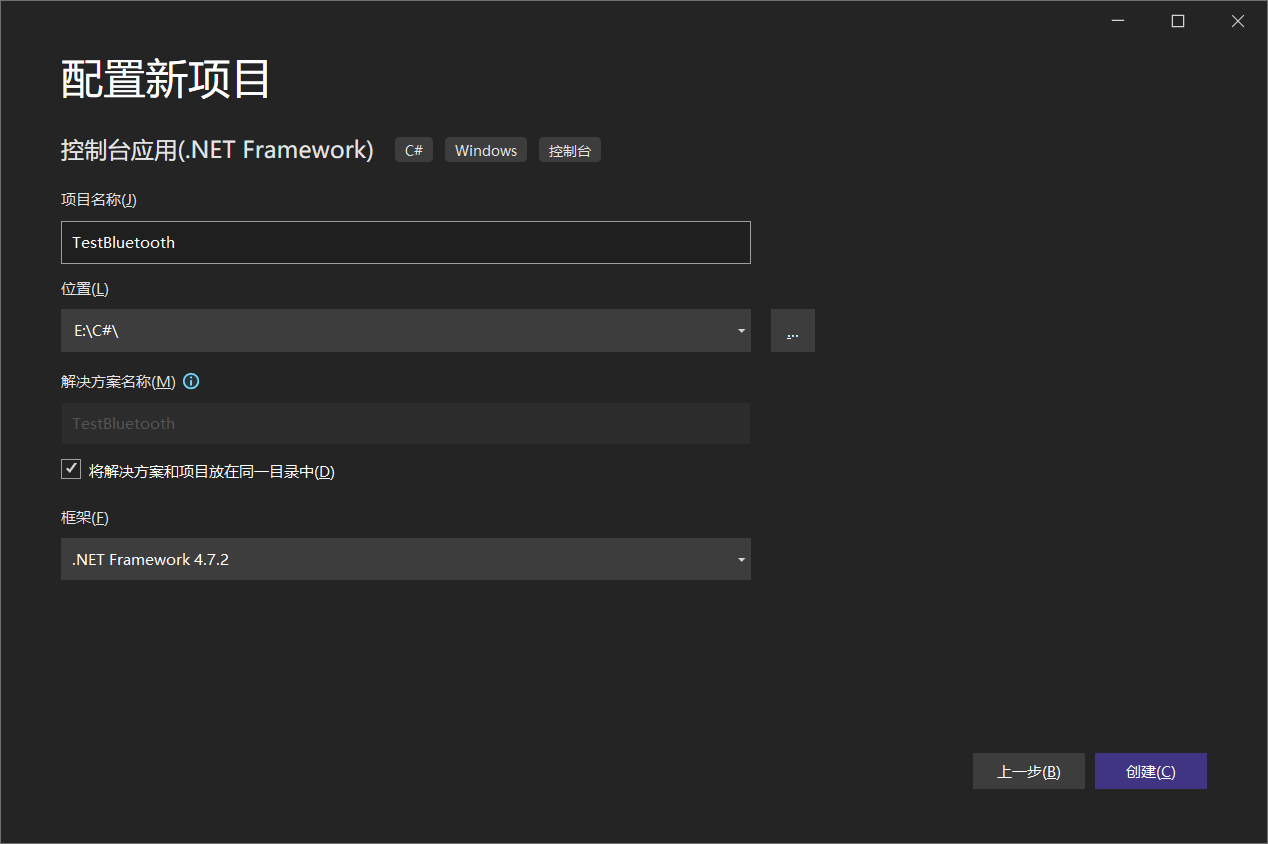
三、安装依赖包
使用VS自带的NuGet下载
工具->NuGet包管理器->管理解决方案的NuGet程序包
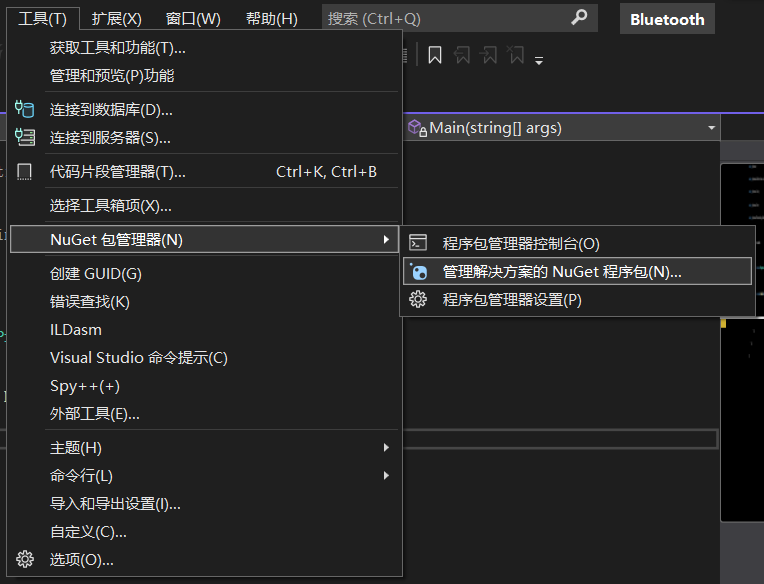
这里有一个坑,添加的包不是InTheHand.NET.Bluetooth,而是32feet.NET
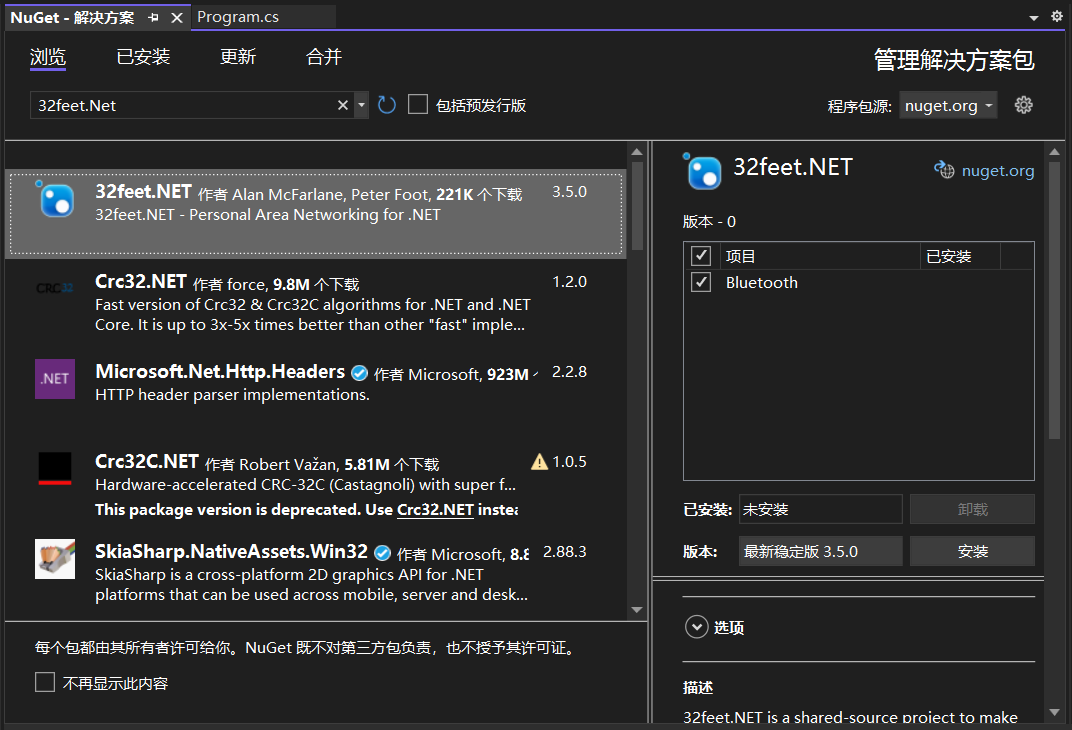
四、编写程序
1.命名空间
1 2 3 4
| using InTheHand.Net; using InTheHand.Net.Bluetooth; using InTheHand.Net.Sockets; using System.IO;
|
2.搜索蓝牙设备
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| bool isFound = false; BluetoothClient client = new BluetoothClient(); BluetoothRadio radio = BluetoothRadio.PrimaryRadio; radio.Mode = RadioMode.Connectable; BluetoothAddress blueAddress = new BluetoothAddress(new byte[] { 0x00, 0x00, 0x00, 0x00, 0x00, 0x00 }); BluetoothDeviceInfo[] devices = client.DiscoverDevices();
foreach (var item in devices) { if (item.DeviceName.Equals("HC-04")) { Console.WriteLine(item.DeviceAddress); Console.WriteLine(item.DeviceName); blueAddress = item.DeviceAddress; isFound = true; break; } }
|
- 蓝牙设备的标识符格式:
与MAC地址类似,由6个字节组成,例如
04-21-11-29-01-D8
- 数组中存放标识符的格式:
从低位开始存。例如上述的地址:
{ 0xD8, 0x01, 0x29, 0x11, 0x21, 0x04}
3.开始连接
1 2 3 4 5 6 7 8 9 10 11 12
| if (!isFound) { Console.WriteLine("没有搜索到设备"); return; } BluetoothEndPoint ep = new BluetoothEndPoint(blueAddress, BluetoothService.SerialPort); Console.WriteLine("正在连接!"); client.Connect(ep); if (client.Connected) { Console.WriteLine("连接成功!"); }
|
4.发送数据
1 2 3 4 5 6
| Stream peerStream = client.GetStream(); Console.ReadKey(); string str = "Hello,world!";
byte[] byteArray = System.Text.Encoding.Default.GetBytes(str); peerStream.Write(byteArray, 0, byteArray.Length);
|
5.完整C#程序
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59
| using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks;
using InTheHand.Net; using InTheHand.Net.Bluetooth; using InTheHand.Net.Sockets; using System.IO;
namespace TestBluetooth { internal class Program { static void Main(string[] args) { bool isFound = false; BluetoothClient client = new BluetoothClient(); BluetoothRadio radio = BluetoothRadio.PrimaryRadio; radio.Mode = RadioMode.Connectable; BluetoothAddress blueAddress = new BluetoothAddress(new byte[] { 0x00, 0x00, 0x00, 0x00, 0x00, 0x00 }); BluetoothDeviceInfo[] devices = client.DiscoverDevices();
foreach (var item in devices) { if (item.DeviceName.Equals("HC-04")) { Console.WriteLine(item.DeviceAddress); Console.WriteLine(item.DeviceName); blueAddress = item.DeviceAddress; isFound = true; break; } } if (!isFound) { Console.WriteLine("没有搜索到设备"); return; } BluetoothEndPoint ep = new BluetoothEndPoint(blueAddress, BluetoothService.SerialPort); Console.WriteLine("正在连接!"); client.Connect(ep); if (client.Connected) { Console.WriteLine("连接成功!");
Stream peerStream = client.GetStream(); Console.ReadKey(); string str = "Hello,world!"; byte[] byteArray = System.Text.Encoding.Default.GetBytes(str); peerStream.Write(byteArray, 0, byteArray.Length); } } } }
|
五、测试
- 1.将HC-04通过CH340与电脑连接
- 2.打开串口调试助手,把波特率调到9600,打开串口
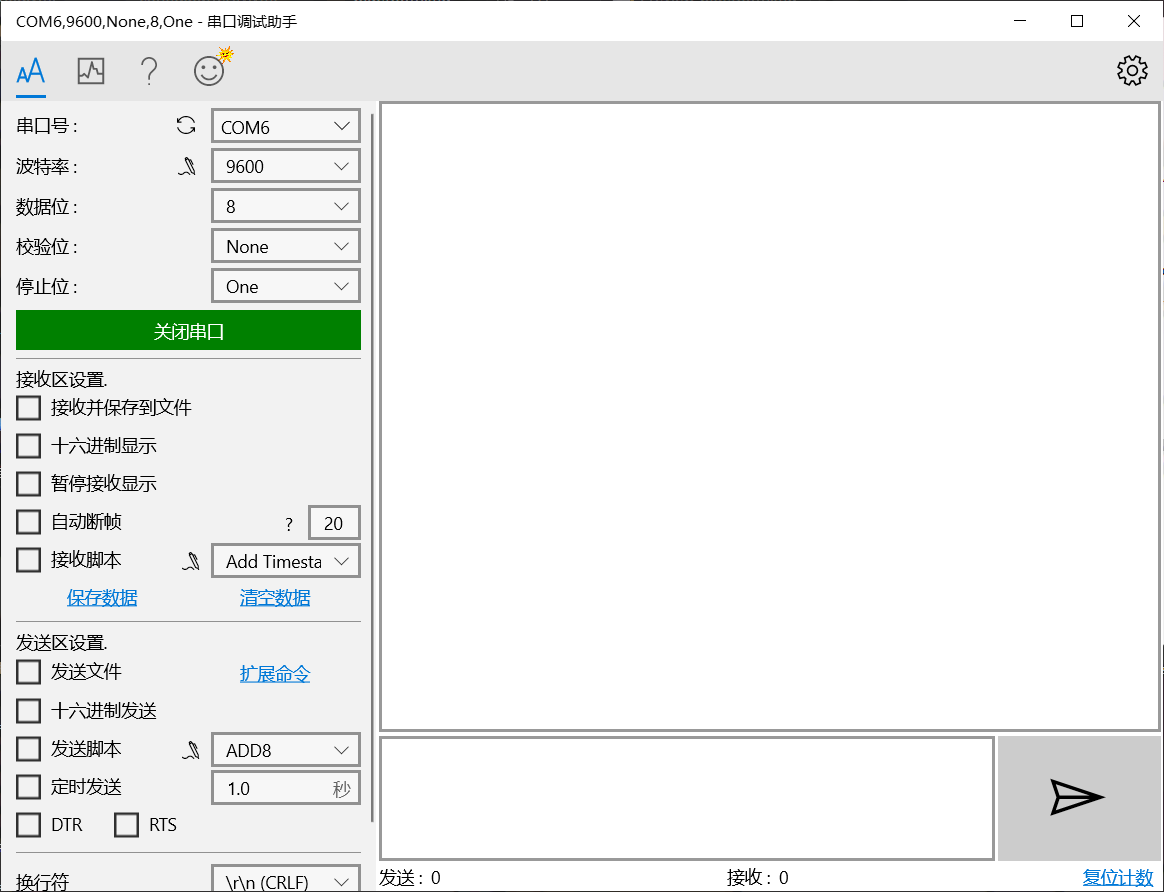
- 3.运行刚刚写的程序,等待连接
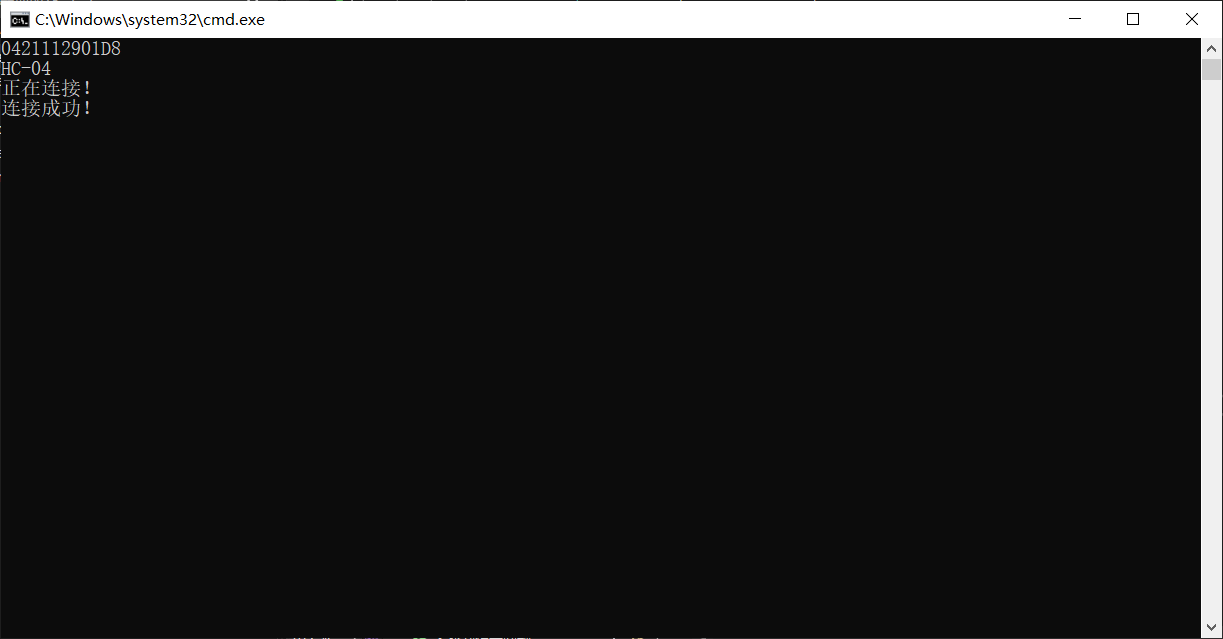
- 连接成功后,按任意键发送,检查串口调试助手
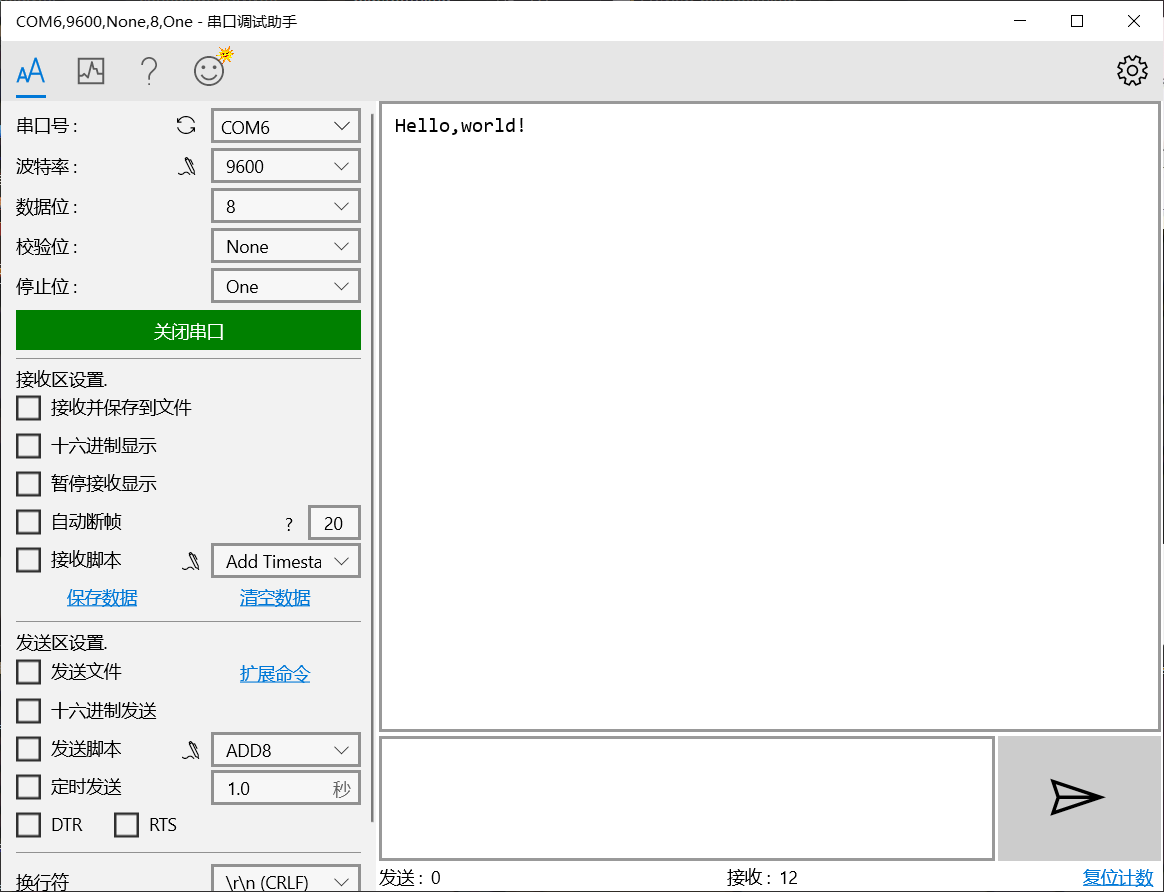
发送成功。
参考链接
不一样的蓝牙连接方式——C#程序实现蓝牙通信
C# 蓝牙开发(经典蓝牙)
C#蓝牙开发 “BluetoothRadio”未包含“PrimaryRadio”的定义